Python Inheritance
Inheritance is a core concept in Object Oriented programming. It allows the programmer to create a hierarchy of classes that share a set of properties and methods.
This is done by deriving a class from another. So one class can inherit the properties of another class. Derived class from Base class.
Creating Base class
Example
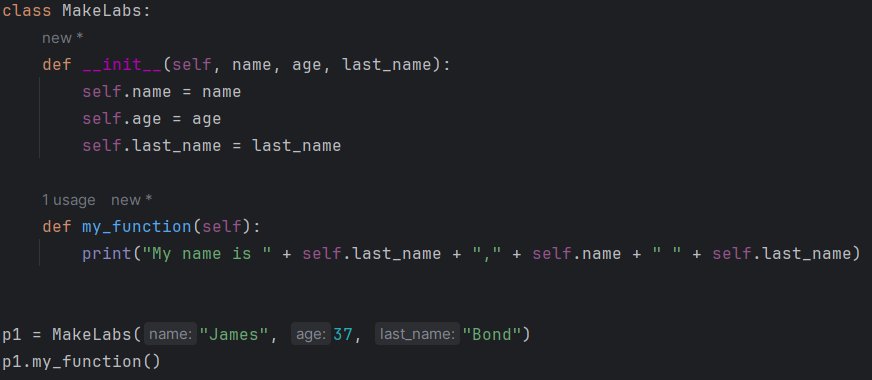
Create Derived class
In order to inherit Derived class (Student) from Base class (MakeLabs), we have to put base class name inside parenthesis.
Example
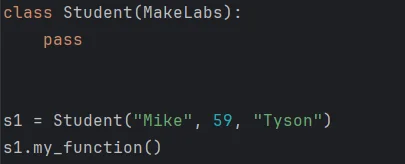
Output
My name is Tyson, Mike Tyson
__init__() Function
__init__() function when added to the derived class won't inherit from parent class' __init__ function.
Example
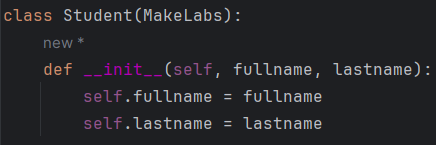
Student class no longer has attributes like name and last_name.
super() Function
super() Function is used to inherit all the methods and properties from its parent class.
Example 1

Output
<__main__.MakeLabs object at 0x0000019883245F10>
Object Methods
Functions that belong to the objects are called methods. Let's create a function in MakeLabs class.
Example
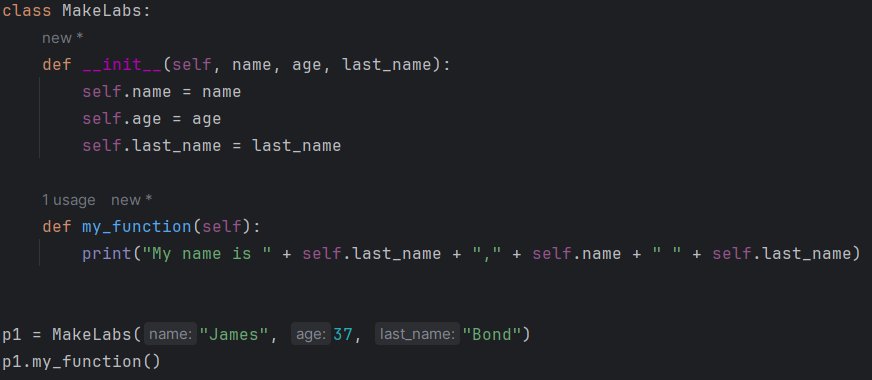
Output
My name is Bond, James Bond
The self parameter
The self parameter references to the current instance of the class. The self parameter is used to access variables that belong to the class.
The name self is a convention to be followed.
Example
When we call p1.my_function() python automatically takes it as MakeLabs.my_function(). This is what self is for.
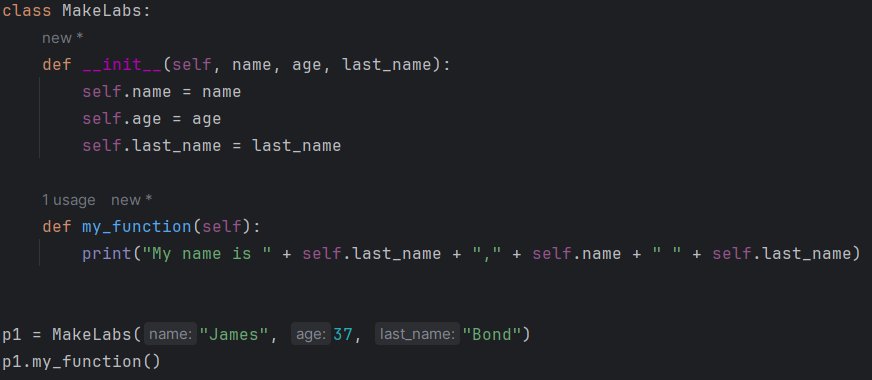