Python Boolean
Boolean Values
In programming, you often need to know if an expression is True or False. Boolean values are the foundation of logical operations and decision-making in programming.
In Python, there are two Boolean values:
- True
- False
Evaluating Expressions
You can evaluate any expression in Python and get one of two answers: True or False. For example:
# Example of Boolean expressions x = 10 y = 5 print(x > y) # Output: True print(x < y) # Output: False
Boolean Operations
Python supports several Boolean operations that can be used to combine Boolean values:
- AND: Returns True if both statements are true.
- OR: Returns True if at least one of the statements is true.
- NOT: Reverses the result, returns False if the result is true.
Examples:
# Example of Boolean operations a = True b = False print(a and b) # Output: False print(a or b) # Output: True print(not a) # Output: False
Common Use Cases
Boolean values are commonly used in conditional statements, such as:
# Using Boolean values in an if statement is_raining = False if is_raining: print("Take an umbrella.") else: print("Enjoy your day!")
Conclusion
Understanding Boolean values and operations is essential for control flow in Python programming. They allow for the creation of more complex logic in applications.
Example Output:

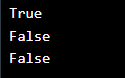