Python RegEx
RegEx Module
Python's re module provides support for regular expressions, which are powerful tools for pattern matching and text manipulation.
Importing re module:
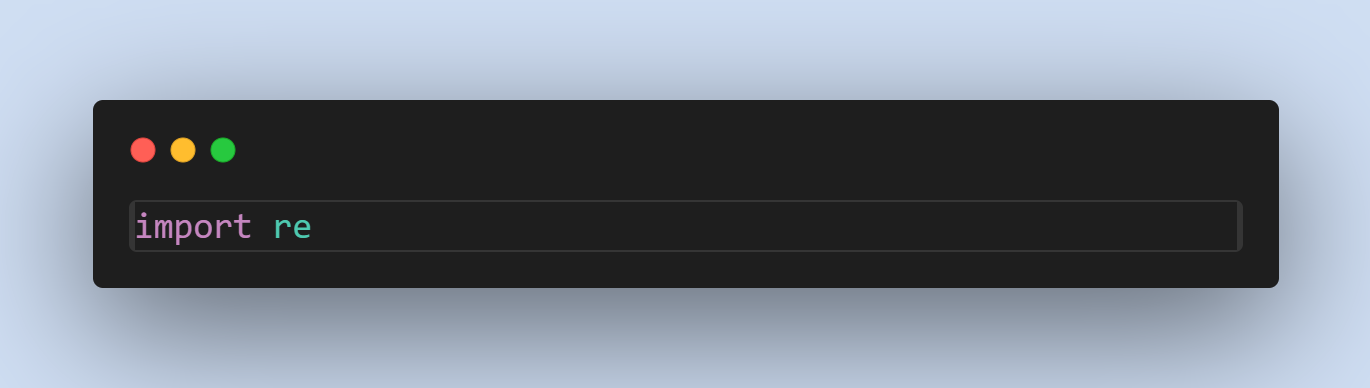
Pattern Matching
Regular expressions are patterns used to match character combinations in strings.
Example:
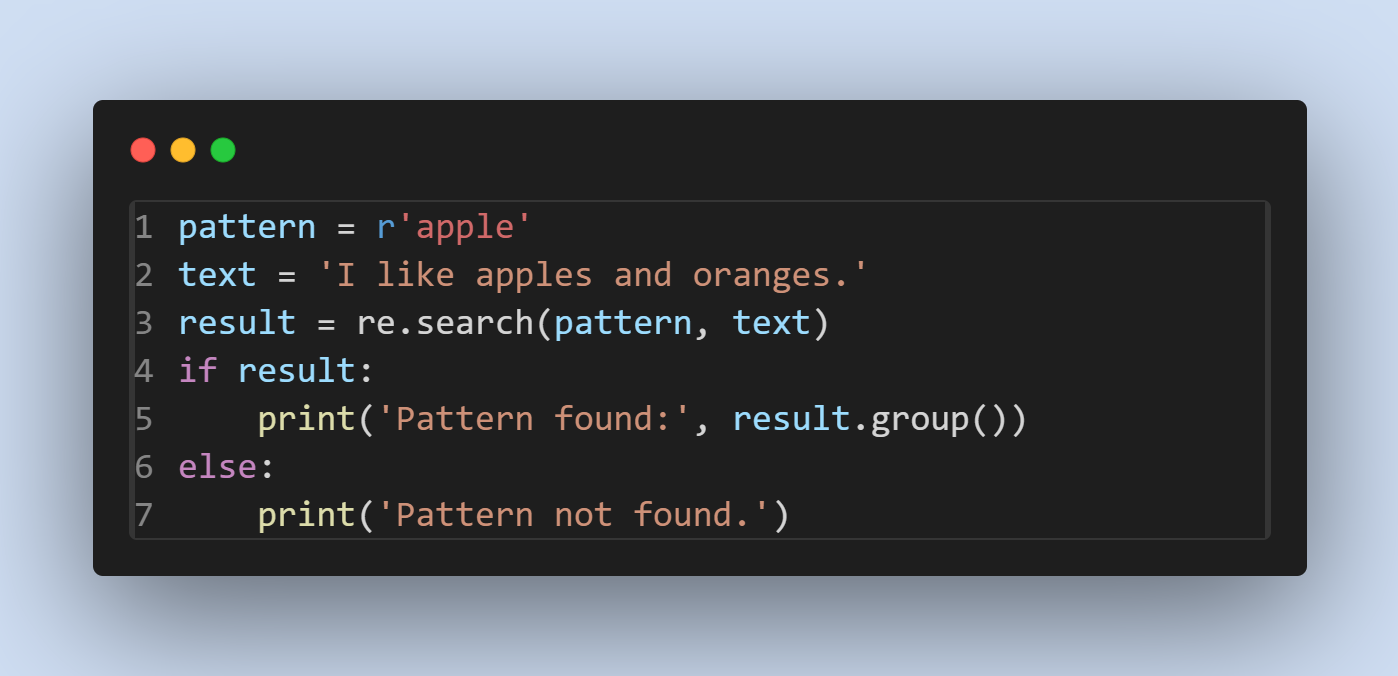
Common Regex Functions
re.search(pattern, string)
: Searches for the first occurrence of pattern in string.re.match(pattern, string)
: Checks if pattern matches at the beginning of string.re.findall(pattern, string)
: Returns a list of all occurrences of pattern in string.re.finditer(pattern, string)
: Returns an iterator yielding match objects for all occurrences of pattern in string.
Regex Patterns
- Metacharacters:
.
(dot): Matches any single character except newline.^
(caret): Matches the start of the string.$
: Matches the end of the string.\d
,\w
,\s
: Match digits, word characters, whitespace, respectively.\b
: Matches word boundaries.
- Quantifiers:
*
,+
,?
: Match 0 or more, 1 or more, 0 or 1 occurrences, respectively.{n}
,{n,}
,{n,m}
: Match exactly n occurrences, n or more occurrences, n to m occurrences, respectively.
- Character Classes:
[abc]
: Matches any of the characters a, b, or c.[^abc]
: Matches any character except a, b, or c.[a-z]
,[0-9]
: Matches any lowercase letter, any digit, respectively.
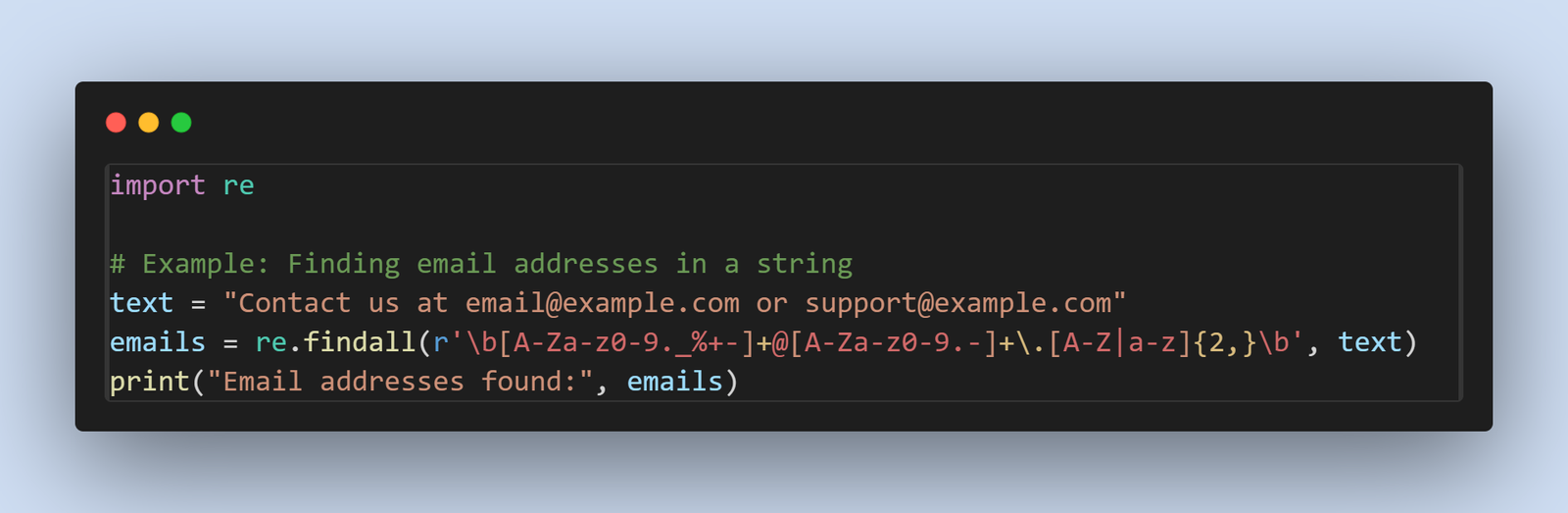