Shyam wants to add the first 100 natural numbers. In mathematics, we would use the sum of series formula or add them 1 by 1 till 100.
Python Loops
While loop in Python
Python has 2 primitive loops
- For loops
- While loops
While loops
While loop is an infinite loop, i.e., a block of code will be in loop till the condition is false.
While loop in Python can help us solving this problem. Let i be 1, the while loop will check if i is less than 6 and add it to sum till it is greater than 6.
When the text condition i.e "i<6" fails the block of code will keep on iterating. The code will stop when i is greater than 6.
Code
i=1 # initializing values
while i < 6: # check condition
print(i) # print the values
i += 1 # value increment
Output
1
2
3
4
5
6
For loops
For loops are used for iterating over an iterable object.
With the for loop, we can execute a set of statements, once for each item in a list, tuple, set, etc.
Code
list=[1,2,3] # list
for i in list: # loop in list
print(i) # print the values
Output
1
2
3
Did you know
You can use loops to automate many day-to-day tasks. Here are some examples:
1. Suppose you are given an Excel file with a list of employees in a company, and you need to create a folder for each of them.
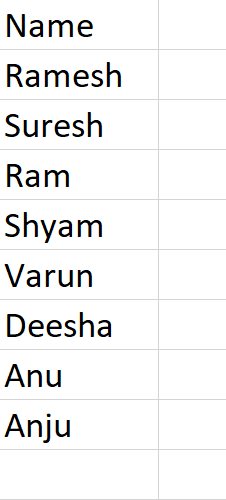
The Excel file contains the names of the employees.
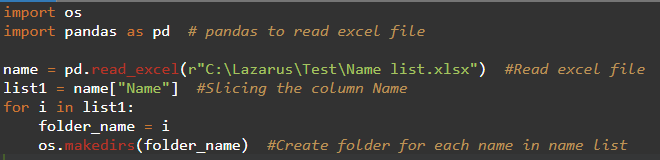
Python script using for loop to create folders
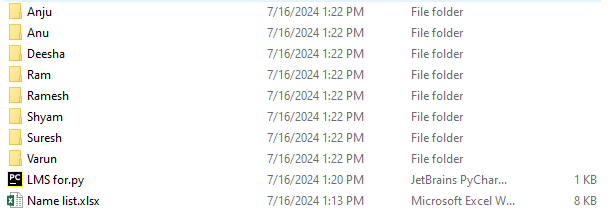
2. Burst shot using Python
Code
import cv2 as cvt
for i in range(10): # for loop
filename = "Image_"+str(i)+".png"
cam = cv.VideoCapture(0)
result, image = cam.read()
if result:
cv.imwrite(filename, image) # Write images
else:
print("No image detected. Please try again")
Output
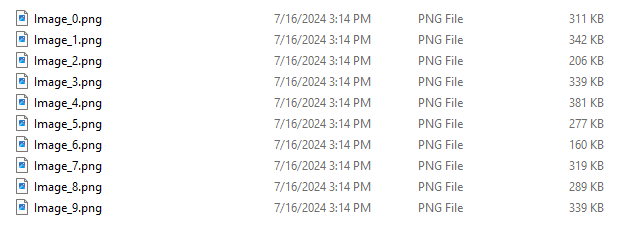