Object Oriented Programming
Python Iterators
An iterator is an object that can be iterated upon, meaning that you can traverse through all the values.
An iterator object contains the methods __iter__() and __next__().
Iterator vs Iterable
List, tuples, dictionaries, strings, and sets are all Iterable objects. They can be converted to iterators. All these have __iter__() methods.
Example
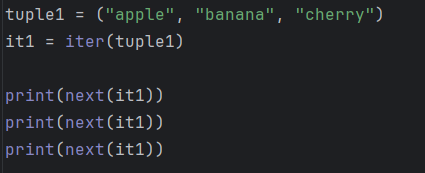
Output
apple
banana
cherry
Looping through Iterator
We use a for loop to iterate through an iterable object. Actually, 'for' creates an iterator object and executes the next() method for each loop.
Example
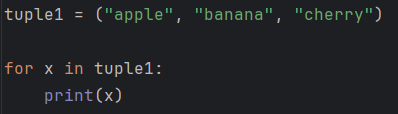
Output
apple
banana
cherry
Create an Iterator
You can create an object/class as an iterator by using __iter__() and __next__() in the object.
__iter__() method acts very similar to __init__() method. It can do initialization but must always return the iterator object itself.
__next__() method allows you to perform operations and must return the next item in the sequence.
Example
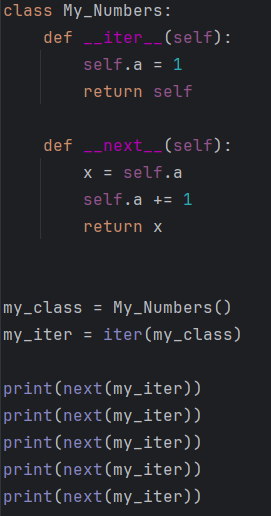
Output
1
2
3
4
5
StopIteration
The iteration can go on forever if there are enough number of next() statements. If the iterator object is used in a loop, it will continue indefinitely.
To stop this iteration, StopIteration is raised at a specific point.
Example
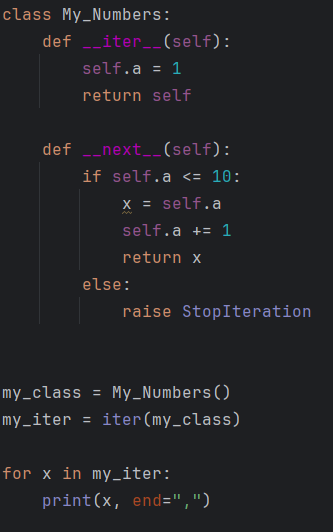
Output
1,2,3,4,5,6,7,8,9,10,